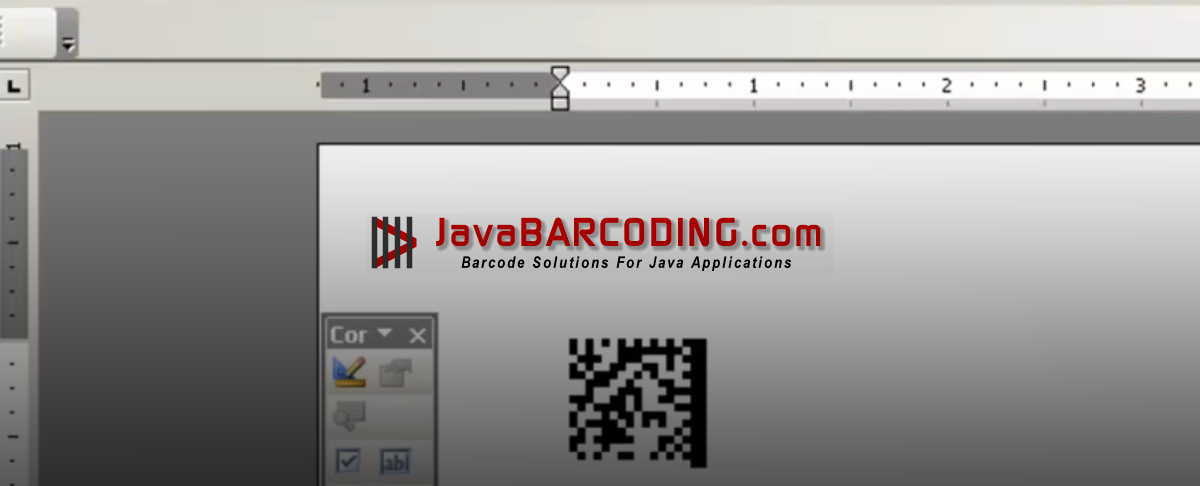
The primary use for Java packages is for printing barcodes to a printer from a web browser. Below is a chart listing the advantages and disadvantages of each type of implementation that displays barcodes on web browsers.
Purchase or Download the IDAutomation Java Barcode Packages.
Applets vs Servlets:
NOTE: IDAutomation no longer recommends Java Applets. The applet element was deprecated in HTML 4.01 and became completely obsolete with the release of HTML5. Additionally, almost all major web browsers have stopped supporting the Java browser plugin.
Servlet Advantages | Servlet Disadvantages |
|
|
Applet Advantages | Applet Disadvantages |
|
|
For other implementations of creating barcodes on web pages, please review the Internet & web pages barcoding FAQ.
* The size of the JAR file can be reduced by about 10K
for applet operation by removing all files in the encoder directory
of the JAR file because these are only used for servlets and graphic
file creation.
Applet Pre-Loading:
Pre-loading the applet on a previous page can reduce the time it takes to create barcodes on web pages using applets. This type of implementation places the JAR file in the browser's cache and allows quick barcode generation - even over slow dial-up lines. The following JavaScript code pre-loads the Linear Barcode Package.
< APPLET
CODE = "IDABarCode.BCApplet"
ARCHIVE = "IDABarCode.jar"
NAME = "PreloadApplet"
WIDTH = 1 HEIGHT = 1 HSPACE = 0 VSPACE = 0 ALIGN = top >
<
/APPLET>
Servlet Source Example
IDAutomation's Servlet is compatible with all browsers and is easy to embed in HTML as an image with the <IMG> tag. See example or view the Servlet demo.
Purchase or Download the Java Barcode Packages now.
Creating secure servlets:
Java Developers that modify the servlet source code provided can accomplish other methods of servlet operation. This may be necessary to prevent the end-user from manipulating the servlet. A working example is provided in the IDAutomationSecureServlet.java file, which is in the source code ZIP file of the linear package.
The following code is an example of the java class library being used as a custom secure servlet:
//*****************************************************************
//
// JAVA Source for com.idautomation.linear; 3.1
//
// Copyright, IDAutomation.com, Inc.
// All rights reserved.
//
// https://www.idautomation.com/java/
//
// NOTICE:
// You may incorporate our Source Code in your application
// only if you own a valid Java Developer License
// from IDAutomation.com, Inc. and the copyright notices
// are not removed from the source code.
//
//*****************************************************************
package com.idautomation.linear;
import javax.servlet.*;
import javax.servlet.http.*;
import java.io.*;
import java.awt.Graphics2D.*;
import java.awt.*;
public class IDAutomationSecureServlet extends HttpServlet
{
/**
* Handle the HTTP POST method by sending an e-mail
*
*
*/
private boolean debug = false;
public void init() throws ServletException {
}
// MODIFY THIS METHOD TO RETRIEVE DATA TO ENCODE,
CREATE THE BARCODE
AND SET THE PARAMETERS
private BarCode getChart(HttpServletRequest request) {
BarCode cb = new BarCode();
cb.code = "SecureServlet";
return cb;
}
// Handle the request
// 1. create barcode
// 2. draw barcode in a Buffered Image
// 3. encode image as GIF or JPEG and send it to the browser
public void doGet(HttpServletRequest request,
HttpServletResponse response)
throws ServletException, IOException
{
PrintWriter out;
ServletOutputStream outb;
//default encoding type
String encode = "jpeg";
if (request != null) {
if (request.getParameter("FORMAT") != null) encode = request.getParameter("FORMAT").toLowerCase();
if (encode.compareTo("gif") != 0) encode = "jpeg";
}
response.setContentType("image/" + encode);
outb = response.getOutputStream();
// avoid caching in browser
response.setHeader("Pragma", "no-cache");
response.setHeader("Cache-Control", "no-cache");
response.setDateHeader("Expires", 0);
try {
// find sizes
int w = 10;
int h = 10;
// get the BarCode
BarCode cb = getChart(request);
if ((request != null) && (request.getParameter("WIDTH") != null) && (request.getParameter("HEIGHT") != null)) {
w = new Integer(request.getParameter("WIDTH")).intValue();
h = new Integer(request.getParameter("HEIGHT")).intValue();
} else {
//a temp image must be created to find the preferred size
cb.autoSize = true;
cb.setSize(170, 90);
java.awt.image.BufferedImage imageTemp = new
java.awt.image.BufferedImage(
cb.getSize().width, cb.getSize()
.height, java.awt.image.BufferedImage.TYPE_BYTE_INDEXED
);
java.awt.Graphics imgTempGraphics = imageTemp.createGraphics();
cb.paint(imgTempGraphics);
cb.invalidate();
w = cb.getSize().width;
h = cb.getSize().height;
imgTempGraphics.dispose();
}
java.awt.image.BufferedImage BarImage = new java.awt.image.BufferedImage
(w, h, java.awt.image.BufferedImage.TYPE_INT_RGB);
java.awt.Graphics2D BarGraphics = BarImage.createGraphics();
if (debug) System.out.println("Size: " + w + " " + h);
cb.setSize(w, h);
cb.paint(BarGraphics);
if (encode.compareToIgnoreCase("gif") == 0) {
// encode buffered image to a gif
// NOTICE - REMARKED NEXT 2 LINES OUT UNTIL UNISYS PATENT EXPIRES
cb.setSize(w, h);
//com.idautomation.linear.encoder.GifEncoder encoder = new com.idautomation.linear.encoder.GifEncoder(BarImage
, outb);
//encoder.encode();
} else
{
// create JPEG image
com.sun.image.codec.jpeg.JPEGImageEncoder encoder = com.sun.image.codec.jpeg.JPEGCodec.createJPEGEncoder(outb);
//increase the JPEG quality to 100%
com.sun.image.codec.jpeg.JPEGEncodeParam param = encoder.getDefaultJPEGEncodeParam(
BarImage);
param.setQuality(1.0 F, true);
encoder.setJPEGEncodeParam(param);
encoder.encode(BarImage, param);
}
} catch (Exception e) {
e.printStackTrace();
}
}
public void doPost(HttpServletRequest request,
HttpServletResponse response)
throws ServletException
{
try {
doGet(request, response);
} catch (Exception e) {
e.printStackTrace();
}
}
}